Mastering Advanced Programming Concepts with Expert Help
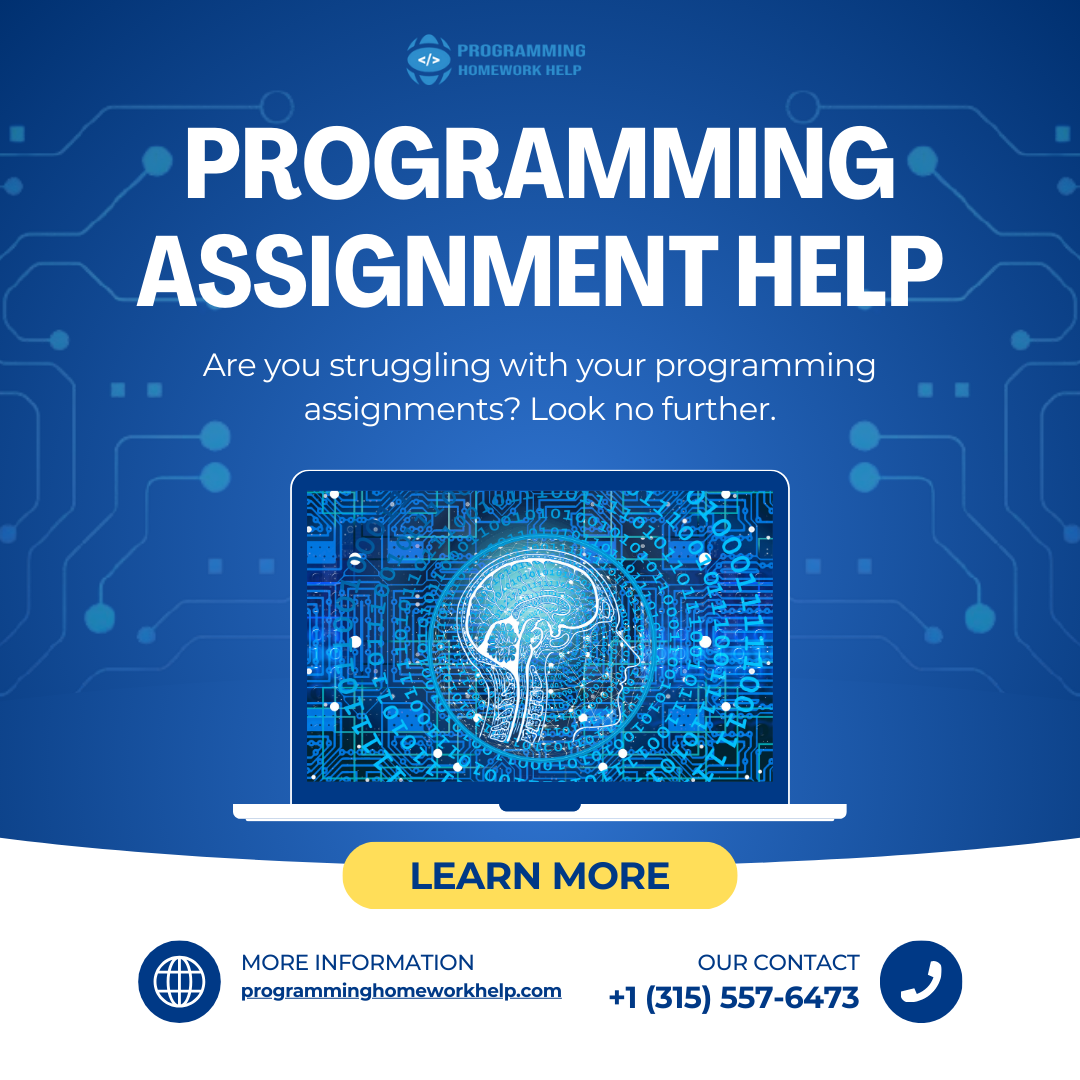
In the world of computer science and software development, mastering advanced programming concepts is a critical step toward achieving academic and professional success. As students delve deeper into their studies, they often encounter complex problems that require a thorough understanding of programming languages, algorithms, and data structures. This is where the value of seeking programming assignment help online becomes evident. With expert guidance, students can navigate these challenges more effectively and achieve a higher level of competence. In this blog, we will explore two master-level programming questions along with their solutions, showcasing how expert assistance can make a significant difference.
The Importance of Advanced Programming Skills
Programming is a field that continuously evolves, with new languages, frameworks, and paradigms emerging regularly. Advanced programming skills are essential for students who aspire to excel in their studies and secure competitive positions in the tech industry. These skills enable students to solve complex problems, optimize code for performance, and contribute to cutting-edge projects. However, mastering these skills often requires more than just attending lectures and reading textbooks. It involves practical experience, critical thinking, and sometimes, a bit of external help.
Master-Level Programming Question 1: Advanced Data Structures and Algorithms
Problem Statement:
You are given a list of intervals representing the start and end times of various events. Your task is to find the maximum number of events that can be attended without any overlap. Each event [start, end] includes both start and end times.
Example:
Output: 3
Explanation: Attend events at intervals [1, 3], [3, 5], and [7, 8]
This problem is a classic example of the interval scheduling maximization problem, which can be efficiently solved using a greedy algorithm. The idea is to always pick the next event that finishes the earliest and is compatible with the previously selected events.
Step-by-Step Solution:
-
Sort the Intervals: Sort the intervals based on their end times.
-
Iterate and Select: Iterate through the sorted intervals, and select an interval if it does not overlap with the previously selected interval.
def max_events(intervals):
# Sort intervals based on end times
intervals.sort(key=lambda x: x[1])
# Initialize variables
max_events_count = 0
last_end_time = float('-inf')
# Iterate through sorted intervals
for interval in intervals:
if interval[0] > last_end_time:
# Select this event
max_events_count += 1
last_end_time = interval[1]
return max_events_count
# Example usage
intervals = [[1, 3], [2, 4], [3, 5], [7, 8]]
print("Maximum number of events that can be attended:", max_events(intervals))
Explanation:
-
Sorting: The intervals are sorted by their end times to ensure that we always consider the earliest finishing events first.
-
Iteration and Selection: By iterating through the sorted intervals and selecting an event if its start time is greater than the last selected event's end time, we maximize the number of non-overlapping events that can be attended.
This algorithm ensures an optimal solution with a time complexity of O(n log n) due to the sorting step, where n is the number of intervals.
Master-Level Programming Question 2: Dynamic Programming and Optimization
Problem Statement:
Given a list of integers representing the lengths of ropes, your task is to find the minimum cost to connect all the ropes into one rope. The cost to connect two ropes is equal to the sum of their lengths.
Example:
Input: ropes = [4, 3, 2, 6]
Output: 29
Explanation: Connect ropes of lengths 2 and 3 (cost = 5), then connect the resulting rope (length = 5) with rope of length 4 (cost = 9), then connect the resulting rope (length = 9) with the remaining rope (length = 6) (cost = 15). Total cost = 5 + 9 + 15 = 29.
Solution:
This problem is a classic example of the minimum cost to connect ropes problem, which can be efficiently solved using a min-heap (priority queue). The idea is to always connect the two shortest ropes first to minimize the cost.
Step-by-Step Solution:
-
Initialize a Min-Heap: Insert all rope lengths into a min-heap.
-
Connect Ropes: While there is more than one rope in the heap, extract the two shortest ropes, connect them, and insert the resulting rope back into the heap. Accumulate the cost of each connection.
import heapq
def min_cost_to_connect_ropes(ropes):
# Initialize a min-heap
heapq.heapify(ropes)
total_cost = 0
# Connect ropes until only one rope is left
while len(ropes) > 1:
# Extract the two shortest ropes
first = heapq.heappop(ropes)
second = heapq.heappop(ropes)
# Calculate the cost to connect them
cost = first + second
total_cost += cost
# Insert the resulting rope back into the heap
heapq.heappush(ropes, cost)
return total_cost
# Example usage
ropes = [4, 3, 2, 6]
print("Minimum cost to connect all ropes:", min_cost_to_connect_ropes(ropes))
Explanation:
-
Min-Heap Initialization: The ropes are inserted into a min-heap to efficiently retrieve the shortest ropes.
-
Connecting Ropes: By always connecting the two shortest ropes, we ensure that the cost is minimized. The total cost is accumulated as we proceed with the connections.
This algorithm ensures an optimal solution with a time complexity of O(n log n) due to the heap operations, where n is the number of ropes.
The Benefits of Seeking Programming Assignment Help Online
Mastering such advanced programming concepts can be challenging, especially when dealing with multiple assignments and tight deadlines. Seeking programming assignment help online offers several advantages:
-
Expert Guidance: Access to experienced professionals who can provide detailed explanations and optimized solutions.
-
Time Management: Efficient use of time by focusing on understanding concepts rather than struggling with difficult problems.
-
Improved Grades: Higher quality assignments lead to better grades and a deeper understanding of the subject matter.
-
Personalized Learning: Tailored assistance that addresses individual learning needs and paces.
At ProgrammingHomeworkHelp.com, we specialize in providing top-notch programming assignment help online. Our team of experts is well-versed in various programming languages and advanced concepts, ensuring that students receive the best possible support. Whether it's solving complex algorithms, optimizing code, or understanding intricate data structures, our services are designed to help students excel.
Conclusion
Advanced programming concepts can be daunting, but with the right guidance, they become manageable and even enjoyable. By seeking programming assignment help online, students can leverage expert knowledge to overcome challenges and achieve their academic goals. The examples provided in this blog demonstrate how expert solutions can simplify complex problems, making it easier for students to grasp and apply advanced programming techniques.
If you're struggling with your programming assignments or simply want to enhance your understanding of advanced concepts, don't hesitate to reach out to us at ProgrammingHomeworkHelp.com. Our experts are ready to provide the assistance you need to succeed in your studies and beyond.
- Art
- Causes
- Crafts
- Dance
- Drinks
- Film
- Fitness
- Food
- الألعاب
- Gardening
- Health
- الرئيسية
- Literature
- Music
- Networking
- أخرى
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness