Mastering Cryptography: Unraveling Complex Challenges with Expert Solutions
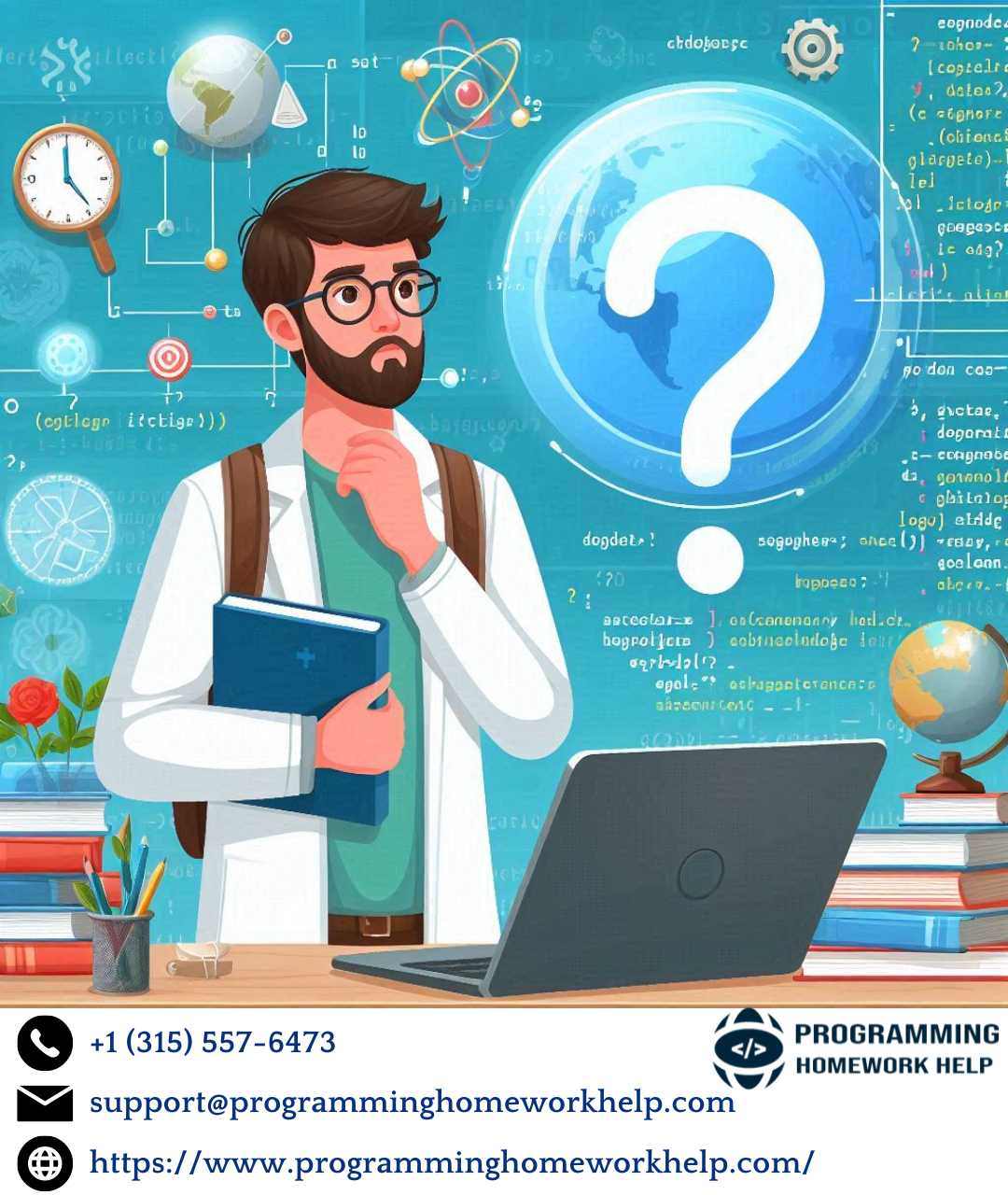
Welcome to ProgrammingHomeworkHelp.com, your trusted destination for online cryptography assignment help. Cryptography, the art of secure communication, presents intricate challenges that require meticulous understanding and expertise to solve. Today, we delve into two master-level cryptography questions, showcasing the depth of our knowledge and the clarity of our solutions. Join us as we unlock the secrets behind these cryptographic conundrums.
Question 1: Caesar Cipher Encryption
You are tasked with implementing a Caesar cipher encryption algorithm in Python. Your function should take two parameters: a string to encrypt and an integer representing the shift value. Implement the function `caesar_cipher_encrypt(plaintext: str, shift: int) -> str` to return the encrypted text.
Solution:
```python
def caesar_cipher_encrypt(plaintext: str, shift: int) -> str:
encrypted_text = ""
for char in plaintext:
if char.isalpha():
shifted_char = chr((ord(char) - ord('a' if char.islower() else 'A') + shift) % 26 + ord('a' if char.islower() else 'A'))
encrypted_text += shifted_char
else:
encrypted_text += char
return encrypted_text
# Example Usage
plaintext = "Hello, World!"
shift = 3
encrypted_text = caesar_cipher_encrypt(plaintext, shift)
print("Encrypted Text:", encrypted_text)
```
Explanation:
The Caesar cipher shifts each letter of the plaintext by a fixed number of positions down or up the alphabet. In our Python implementation, we iterate through each character of the plaintext. If the character is a letter, we calculate the new shifted character based on the ASCII values. We handle both uppercase and lowercase letters separately, ensuring the case is preserved. Non-alphabetic characters remain unchanged.
Question 2: Vigenère Cipher Decryption
You are given an encrypted message using the Vigenère cipher along with the keyword used for encryption. Implement a Python function `vigenere_cipher_decrypt(ciphertext: str, keyword: str) -> str` to decrypt the message.
Solution:
```python
def vigenere_cipher_decrypt(ciphertext: str, keyword: str) -> str:
decrypted_text = ""
keyword = keyword.lower()
key_length = len(keyword)
for i, char in enumerate(ciphertext):
if char.isalpha():
key_index = i % key_length
shift = ord(keyword[key_index]) - ord('a')
decrypted_char = chr((ord(char) - ord('a' if char.islower() else 'A') - shift) % 26 + ord('a' if char.islower() else 'A'))
decrypted_text += decrypted_char
else:
decrypted_text += char
return decrypted_text
# Example Usage
ciphertext = "Jevpq, Wyvnd!"
keyword = "cipher"
decrypted_text = vigenere_cipher_decrypt(ciphertext, keyword)
print("Decrypted Text:", decrypted_text)
```
Explanation:
The Vigenère cipher is a polyalphabetic substitution cipher that uses a keyword to shift letters in the plaintext by different amounts. Our Python function decrypts the ciphertext by iterating through each character, applying the reverse shift based on the keyword. We calculate the shift for each letter dynamically based on the position in the keyword. Non-alphabetic characters remain unchanged.
Conclusion
Mastering cryptography requires a deep understanding of various encryption and decryption techniques. Through these expert solutions, we've demonstrated the effectiveness of our approach in tackling complex cryptographic challenges. Whether you're a student seeking guidance or a cryptography enthusiast, ProgrammingHomeworkHelp.com is your ultimate destination for unlocking the secrets of cryptography. Stay tuned for more insightful content and don't hesitate to reach out for personalized assistance with your cryptography assignments.
- Art
- Causes
- Crafts
- Dance
- Drinks
- Film
- Fitness
- Food
- Jeux
- Gardening
- Health
- Domicile
- Literature
- Music
- Networking
- Autre
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness